ListView stands as a foundational component for displaying scrollable lists in Android apps. Its versatility and ease of implementation make it essential for efficient user interfaces.
Whether you are going to get help from the best mobile app development company in Dallas or working yourself, this guide help you go through and even implement successfully.
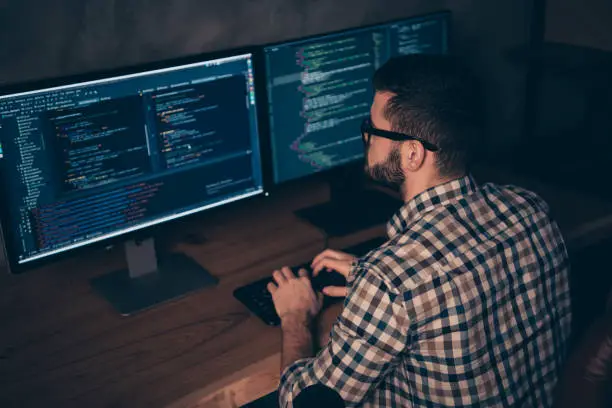
Getting Started!
To implement ListView, start by defining the ListView in your layout XML file or create it programmatically. Link it with an adapter to manage the data displayed within. Let’s go through the top tips that top mobile app development companies implement.
Choosing the Right Adapter:
Selecting the appropriate adapter is crucial to implementing ListView in Android applications. It acts as a bridge between data sources and the ListView, defining how data is presented to users.
ArrayAdapter: Ideal for Simple Data Display
ArrayAdapter is straightforward and perfect for displaying simple data structures like arrays or lists. It efficiently converts data into view items without complex customization.
BaseAdapter: Customization and Flexibility
BaseAdapter offers more control and customization options. It serves as a base class for creating custom adapters. It provides greater flexibility for complex data structures and customized views.
CursorAdapter: Linking with Database Results
CursorAdapter integrates seamlessly with database queries. It binds data retrieved from a cursor (database query result) to the ListView efficiently. It’s good for handling database-backed lists.
SimpleCursorAdapter: Effortless Database Data Binding
SimpleCursorAdapter simplifies binding data from the database to the UI elements. It reduces boilerplate code for common use cases and is suitable for simpler data-binding scenarios.
Custom Adapter: Tailoring to Unique Needs
Creating a custom adapter offers complete control over the data and view rendering process. It is ideal for intricate data structures or when customizing views beyond the capabilities of built-in adapters.
Which One is Best for You?
Consider the complexity of your data, the level of customization required for views, and the data source itself when selecting an adapter. For basic data structures, ArrayAdapter serves well, while BaseAdapter caters to more intricate needs.
If you’re working with databases, CursorAdapter or SimpleCursorAdapter streamline data integration. For intricate UI designs, crafting a custom adapter might be the best fit.
Populating the ListView:
To implement ListView in Android, populate it with data. It is a crucial step for a functional and user-friendly interface.
Data Source Selection:
Begin by determining the data source! This could be an array, a database query result, or any collection of items that need to be displayed.
Adapter Setup:
Next, create an adapter. It can be ArrayAdapter or BaseAdapter based on the nature and complexity of the data source.
Linking Data and ListView:
Set the adapter to the ListView using the setAdapter() method. This establishes the connection between the data source and the visual representation in the ListView.
Customizing the Display:
Customize the display of data within the ListView by defining how each item in the data source will be presented. This can involve creating custom layouts or modifying the default layout provided by the adapter.
Binding Data:
Use the adapter’s getView() method to bind the data to the corresponding views within the ListView.
Refreshing and Updating:
For dynamic data or when changes occur, update the dataset associated with the adapter. After that, call notifyDataSetChanged() to refresh the ListView and reflect the changes instantly.
Performance Considerations:
Optimize performance by employing techniques like view recycling. Thoroughly test the populated ListView across different devices and screen sizes.
Enhancing User Interaction:
User interaction is pivotal in delivering an engaging ListView experience in Android applications. Here’s how to elevate user engagement:
ItemClickListeners:
Implement setOnItemClickListener to respond to item clicks. It offers users seamless navigation to detailed views or performs specific actions based on selections.
ItemLongClickListeners:
Utilize setOnItemLongClickListener to enable extended functionalities or context-based actions upon a long press. It enhances user control over items in the list.
Visual Feedback:
Provide visual cues using animations or changing item backgrounds upon selection. This thing offers users immediate feedback on their interactions for a more intuitive experience.
Customizing Click Events:
Tailor the click events to handle various user actions like double clicks or swipes.
Contextual Actions:
Implement contextual actions like contextual menus or pop-ups triggered by user interactions. Implementing this offers additional functionalities or choices based on specific item selections.
Feedback Handling:
Address user feedback promptly by incorporating loading indicators, progress bars, or notifications during data updates or when fetching information for seamless interaction.
Accessibility Features:
Ensure accessibility by providing content descriptions for items. This thing enables screen reader compatibility and facilitates interaction for users with disabilities.
Error Handling:
Handle errors gracefully by communicating errors or empty states effectively. This thing guides users and maintains a smooth user experience even during unexpected situations.
Optimizing Performance:
Optimizing performance in ListView implementation is crucial for creating responsive and efficient Android applications. Several strategies ensure smooth functionality and enhance the user experience.
ViewHolder Pattern:
Employ the ViewHolder pattern to enhance scrolling performance. Reusing views within the ListView significantly reduces the overhead of inflating new views and optimizes memory usage.
View Recycling:
Implement view recycling to reuse off-screen views. As the user scrolls, views that are no longer visible are recycled to display new data. This reduces memory consumption and improves scrolling speed.
Lazy Loading:
Implement lazy loading or pagination for large datasets. Load data dynamically as needed and prevent excessive memory usage to ensure faster initial rendering.
Image Optimization:
Optimize their loading using libraries like Picasso or Glide when dealing with images. These libraries handle image caching, resizing, and asynchronous loading to improve performance significantly.
Batching Updates:
When updating the ListView, batch changes to the adapter are made judiciously using notifyDataSetChanged(). Minimize unnecessary updates to enhance performance.
Consider RecyclerView:
For more complex list UIs or when working with larger datasets, consider using RecyclerView instead of ListView. RecyclerView offers better performance optimizations and flexibility.
Asynchronous Loading:
Fetch data asynchronously from external sources or databases. Implement background threads or AsyncTask to prevent UI blocking and ensure a smoother user experience.
Proper Resource Handling:
Dispose of resources properly. Release memory, unregister listeners, and close cursors when they are no longer needed.
Testing and Refinement:
Testing your ListView implementation is crucial to ensure a seamless user experience. Here’s a step-by-step approach:
Functional Testing:
Begin by examining basic functionality. Ensure that the ListView displays the intended data correctly, scrolls smoothly, and responds accurately.
Performance Evaluation:
Assess performance metrics such as scrolling speed, especially with larger datasets. Optimize performance using techniques like view recycling and ViewHolder pattern for smoother scrolling.
Edge Cases Handling:
Check for edge cases, such as empty lists or extreme data sizes. Ensure the ListView gracefully handles scenarios like no data to display or a vast amount of information without crashing or slowing down.
User Experience Refinement:
Solicit feedback or conduct usability tests to understand user interactions. Refine the ListView based on user input, making adjustments to improve usability and intuitiveness.
Compatibility and Backward Support:
Consider backward compatibility to cater to a broader user base without sacrificing functionality or appearance.
Localization and Accessibility:
Validate ListView functionality in various languages and ensure accessibility features work seamlessly, including screen readers and text size adjustments.
Security and Error Handling:
Implement error handling mechanisms for potential issues like network errors when fetching data. Prioritize data security and implement secure coding practices.
Documentation and Maintenance:
Document your ListView implementation comprehensively for future reference or team collaboration. Maintain the codebase regularly for updates and improvements.
Conclusion:
Mastering ListView implementation is fundamental for creating intuitive, user-friendly Android applications. Its adaptability and simplicity empower developers to craft dynamic and responsive interfaces effortlessly.
Implementing ListView in Android opens doors to crafting engaging user interfaces, and understanding its nuances is essential for developers aiming to create compelling apps.